Getting Started
Please Note The following instructions are mandatory to ensure app compliance. Please make sure to follow the entire guide before submitting your app to Outbrain QA.
Intro
This document describes the Outbrain mobile SDK for the iOS platform. It is intended for iOS mobile app developers who want to integrate the Outbrain product into their code. The document describes the main Outbrain interface functions.
Compatibility and Requirements
The Outbrain SDK is a framework, and is compatible with iOS 10.3 and upward. In addition, you will need to add AdSupport.framework
and SystemConfiguration.framework
.
Outbrain SDK | Requirements |
---|---|
Min SDK | iOS 14.0 |
Build SDK | iOS 17.0 |
Languages | Objective-C, Swift |
Devices | Any iOS compatible device: iPhone, iPad, etc |
SDK Size | OutbrainSDK adds about 100KB to your iOS release app. |
Architectures | i386, x86_64, armv7, arm64 |
Outbrain Application Review
A finalized build must be sent to Outbrain QA at least one week (5 working days) prior to your anticipated release date. We may request changes that will require additional dev resources and could delay your release.
We reserve the right to remove our recommendations or restrict the app from generating Outbrain revenue if required changes are not incorporated prior to release. Builds can be submitted via TestFlight or HockeyApp according to the following table:
Publisher Location | |
---|---|
US & LatAm | usqa@outbrain.com |
EU, APAC & EMEA | ilqa@outbrain.com |
Your Account Manager can provide more details.
Recommendation Display Guidelines and Limitations
The recommendations that Outbrain provides are time-sensitive. Recommendations are intended for specific users at specific times, and any caching or delay in their presentation will have a negative impact on their performance (and the user experience).
The following limitations and guidelines must be applied to the Outbrain recommendations’ display:
- Storing/caching recommendations with the intent of delaying their presentation on the page is prohibited.
- Co-mingling of Outbrain recommendations with other content links within the same container is prohibited, unless mutually agreed upon between the client and Outbrain.
- Altering or replacing an Outbrain recommendation’s text or image is prohibited.
- All paid recommendations must be uniquely labeled in a manner that can be reasonably associated with the relevant content, as mutually agreed upon between the client and Outbrain.
Integrating with the Outbrain SDK
There are many options to integrate with Outbrain SDK:
- Directly download the XCFramework and add it to your Xcode project.
- Swift Package Manager
- Podfile (Cocoapods)
Please follow the instructions on Download Links
Initializing the Outbrain SDK
The Outbrain Interface
The Outbrain interface is the main interface to the Outbrain SDK. Your app calls its methods in order to initialize the SDK, request recommendations and report clicks.
Partner Key
You will need to register your app’s Outbrain configuration once during the initialization of your app, before calling any other Outbrain method. You can do this by calling Outbrain’s initializeOutbrainWithPartnerKey
method. This method takes a single appKey parameter, which is a string that contains the partner key
you’ve received from your Outbrain account manager.
Here is an example of how to call initializeOutbrainWithPartnerKey:
Objective C
[Outbrain initializeOutbrainWithPartnerKey:@"MyPartnerKey"];
Swift
Outbrain.initializeOutbrain(withPartnerKey: "MyPartnerKey")
You can check whether SDK is already initlized by calling:
Objective C
[Outbrain SDKInitialized];
Swift
Outbrain.sdkInitialized()
Working in Test Mode
While you are developing your app and debugging the integration with Outbrain, you must configure the SDK to work in test mode. This prevents Outbrain from performing operational actions such as reporting and billing, for clicks that were not made by your app’s users.
Here is an example:
Objective C
#if DEBUG
[Outbrain setTestMode:YES];
#endif
Swift
#if DEBUG
Outbrain.setTestMode(true)
#endif
During the development and testing phase, call setTestMode during your app’s initialization, but remember to remove this call before submitting your app to the app store.
Note: Please use test mode only during development and testing phases. Default value is “false”.
Simulate Location (Geo)
When in testMode
it’s also possible to simulate location in order for Outbrain servers to return recs according to set location. An example use-case for this would be app developers in India trying to simulate recs for UK audience.
Here is an example for setting US location
Objective C
#if DEBUG
[Outbrain testLocation:@"us"];
#endif
Swift
#if DEBUG
Outbrain.testLocation("us")
#endif
Apple third-party SDK requirements
Privacy Manifest File (PrivacyInfo)
PrivacyInfo.xcprivacy
is included in all iOS SDK versions starting from 4.9.0
Following Apple new guidelines:
"Apps and third-party SDKs — should contain a privacy manifest file, named PrivacyInfo.xcprivacy. The privacy manifest is a property list that records the types of data collected by your app or third-party SDK, and the required reasons APIs your app or third-party SDK uses."
Signatures for SDKs
SDK signature
is included in all iOS SDK versions starting from 4.30.0
Following Apple Signatures for SDKs guidelines:
Now with signatures for SDKs, when you adopt a new version of a third-party SDK in your app, Xcode will validate that it was signed by the same developer, improving the integrity of your software supply chain.
Outbrain SDK is signed with Outbrain Apple Developer ID. This will ensure that the SDK is not tampered with and that it is from a trusted source.
GDPR Support
Outbrain SDK supports the IAB guidance and solutions for the requirements of the General Data Protection Regulation.
In February 2017, the IAB Europe assembled parties representing both the supply and demand sides of the digital advertising ecosystem, to work collectively on guidance and solutions to the requirements of the General Data Protection Regulation (GDPR). That working group is known as the GDPR Implementation Working Group (GIG). One of the sub-groups within the GIG was tasked with developing guidance on consent as a legal basis for processing personal data. Out of that effort, an additional working group was formed to develop a technical solution to the challenge of obtaining and disseminating consumer consent to the various parties relying on it as a legal basis of processing personal data.
IDFA changes (optional)
One of iOS14 main changes in regards to privacy and tracking is the decision to disable access to the user IDFA by default (and postponed the enforcement to early 2021 since then). Thus, as of iOS 14 in order to access the IDFA the app developer must as for the user explicit permission.
See code example below: (plist file edit is required as well)
if #available(iOS 14, *) {
ATTrackingManager.requestTrackingAuthorization { authStatus in
print("user authStatus is: \(authStatus)")
print("advertisingIdentifier: \(ASIdentifierManager.shared().advertisingIdentifier)")
}
}
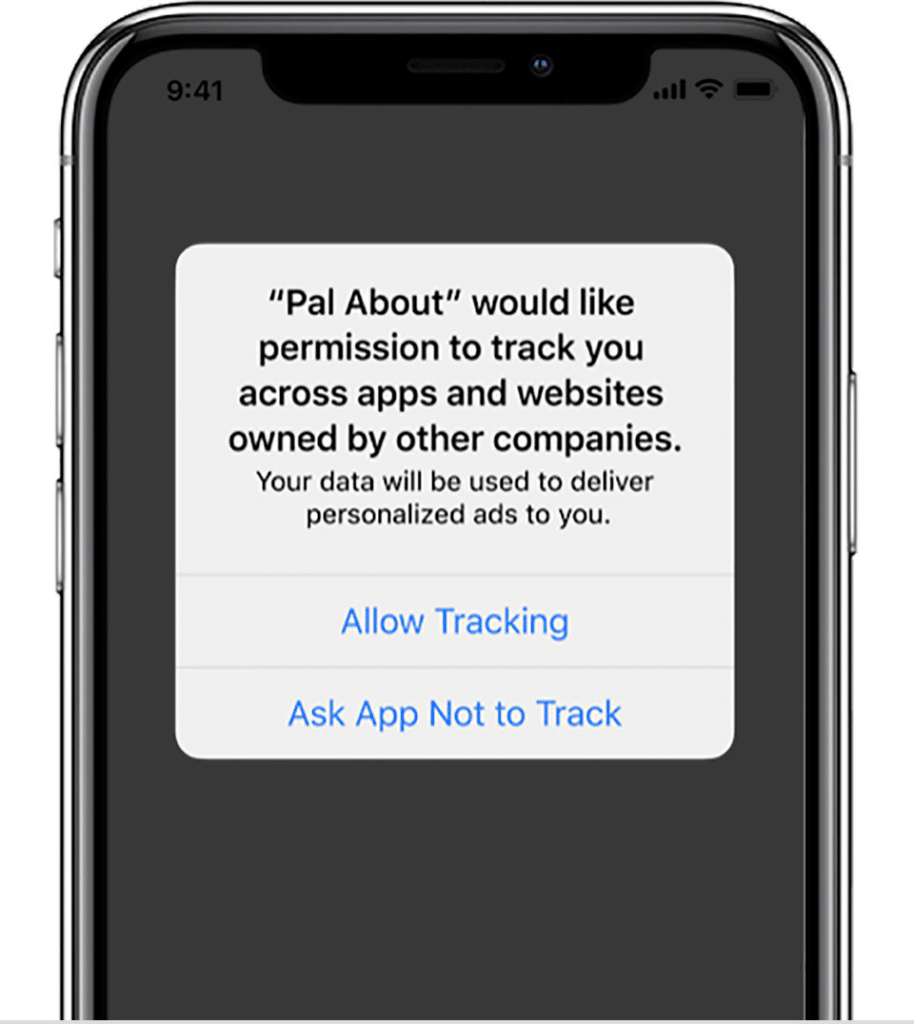